Working with Referrer Pages
A referrer page is the page that the user was viewing before the current page, or, in other words, the page the user came from. You may wish to provide a message to the user and mention the referrer page, as shown in Figure 2.12. One way to use this technique is when you have set up an affiliate program with another site. You can detect the referring site and bounce the visitor to a special welcoming page. Script 2.14 has the simple HTML, and you'll find the JavaScript in Script 2.15.
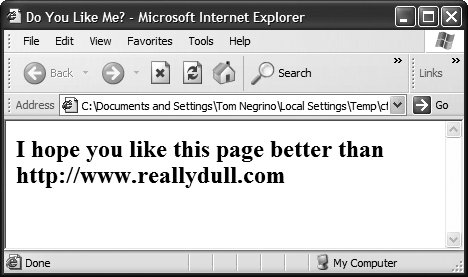
Script 2.14. The empty <h2> tag has an id for use by the referrer page JavaScript.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Welcome to our site</title>
<script src="script09.js" type="text/javascript" language="javascript">
</script>
</head>
<body bgcolor="#FFFFFF">
<h2 id="referrerMsg">
</h2>
</body>
</html>
|
Script 2.15. The referrer code.
window.onload = writeMessage;
function writeMessage() {
if (document.referrer != "") {
document.getElementById("referrerMsg"). innerHTML = "I hope you like this page
better than " + document.referrer;
}
}
|
To display the referrer page:
1. |
<h2 id="referrerMsg"></h2>
This is in Script 2.14. As in past examples, it's an empty headline tag with a specified id.
| 2. |
if (document.referrer != "") {
Script 2.15 is easy to understand. If the referrer page, represented here by document.referrer, is not empty, then continue through the script. The document.referrer object could be empty if the user hadn't visited any other page before yours, i.e., if they opened their browser directly to your page.
| 3. |
document.getElementById ("referrerMsg").innerHTML = "I hope you like this page better than
" + document.referrer;
If the document.referrer object isn't empty, then write out a message to the document with a text string, concatenating the document.referrer to the end of the string.
|
Tips
This technique can also be harnessed to trigger different actions depending on what the referrer page was. You could find out the domain of the referrer page and then serve up something special for users who came from a particular domain. When this book went to press, the URL in Figure 2.12 had a humorous destination. Try it and see.
If you work with JavaScript for any length of time, you may start to feel overwhelmed by the amount of terminology that gets thrown aroundand much of it, at its root, is about what JavaScript is and isn't. Here's a quick rundown of where we stand on these burning issues (and if you don't think they're burning issues, hang out with some scripters for a while!):
JavaScript: While officially this term is owned by AOL (via Netscape), it's commonly used to cover all JavaScript-like scripting technologies such as Microsoft's JScript. We'll continue that trend in this book. DHTML: This stands for Dynamic HTML, but in real life, what that actually means depends on who's doing the talking. The DOM Scripting Task Force of the Web Standards Project defines DHTML (webstandards.org/action/dstf/definitions/) as "...an outdated scripting technique that is mainly characterized by the changes it makes to the style properties of certain elements, and by the use of the browser-specific DOMs document.layers and document.all." On the other hand, Myspace.com (in Figure 2.13) says that when you're editing your profile, "You may enter HTML/DHTML or CSS in any text field. JavaScript is not allowed," leading their huge user base to believe that DHTML has nothing to do with scripting (and is possibly interchangeable with XHTML).
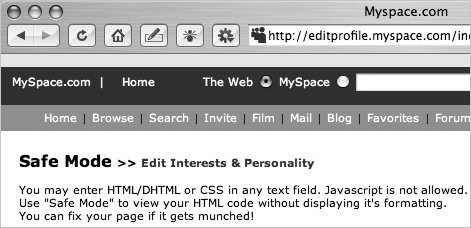
Our opinion is that DHTML is much too confusing and imprecise a term, so we no longer use it. If you come across anyone who does, make sure they define it. DOM scripting: An approach to scripting Web pages using JavaScript, in which the code only modifies pages via manipulation of the W3C DOM (that is, no use of proprietary, non-standard, or deprecated properties). When Scripts 2.10 and 2.13 refer to document.getElementById("redirect").onclick, that's DOM Scripting in action. Unobtrusive scripting: An approach to scripting Web pages using JavaScript, in which the behavior of the Web page is kept separate from its contentthat is, the HTML is in one file, and the JavaScript is in another. As a best-practices recommendation, it's comparable to the split between HTML and CSS, where the presentation (CSS) is in one file and the content (HTML) is in another. Additionally, the term "unobtrusive scripting" is used when code is written such that visitors without JavaScript (or with less-capable browsers) get all the functionality of a site, just with a less-rich user experience. Scripts 2.10 and 2.13 are also examples of unobtrusive scripting, in that you don't need JavaScript in order to click the links, but you'll have a richer experience when you do.
Throughout this book, we use a variety of scripting techniques. While we recommend the unobtrusive scripting approach wholeheartedly (and we try to demonstrate it whenever possible), we also know that you, as a budding scripter, will frequently need to be able to understand and support older, less-rigorously written code. And finally, this being the real world, we also know that sometimes the simplest way to hammer in a nail is to grab a rock and pound the nail into the wall. This, for instance, is why we used innerHTML back in Script 2.3, even though it's not part of the W3C DOM.
|
|