Form Event Handling
You'll want to use form event handling mainly for validating forms. With the events listed below, you can deal with just about any action the user takes with forms.
The onsubmit event
The onsubmit handler (as seen in Chapter 7) is triggered when the user clicks the Submit button to complete a form. In addition, depending on the browser, it can also be triggered when a user exits the last text entry field on a form. If a script contains an onsubmit handler, and the result of the handler is false, the form will not be sent back to the server.
The onreset event
The onreset handler is triggered when the user clicks the Reset button (if one is present) on a form. This can be handy if your form has default values that are set when the page loadsif the user clicks Reset, you'll need to handle this situation with a script that resets the default values dynamically.
The onchange event
As shown in Script 7.2, the onchange event handler is triggered when the user changes a form field. This can be used to verify they entered information immediately, or to respond to the user's choice before they click the Submit button.
The onselect event
The onselect handler is triggered if the user selects text in either an input or a textarea form field.
The onclick event
While the onclick handler is mentioned above under "Mouse Events," it's listed here again because it's most commonly used when dealing with forms. This event is triggered when the user clicks a check box or radio button, as in Script 7.13. Script 2.10 also uses the onclick handler; in that case, it allows a single link to do one action for JavaScript-enabled browsers and another, entirely different action, for browsers without JavaScript.
The onblur event
While onblur can be used for browser windows (as shown above), it's more common for it to be used in forms. Scripts 9.15 and 9.16 show the onblur handler being used to force the user to enter data into a field.
Script 9.15. This HTML creates the simple form.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Requiring an entry</title>
<script language="Javascript" type="text/javascript" src="script08.js">
</script>
</head>
<body bgcolor="#FFFFFF">
<form action="#">
<h3>
Email address: <input type="text" class="reqd" /><br /><br />
Name (optional): <input type="text" />
</h3>
</form>
</body>
</html>
|
Script 9.16. The onblur handler can be used in forms to trigger actions when the user leaves a field.
window.onload = initForm;
function initForm() {
var allTags = document.getElementsByTagName ("*");
for (var i=0; i<allTags.length; i++) {
if (allTags[i].className.indexOf ("reqd") > -1) {
allTags[i].onblur = fieldCheck;
}
}
}
function fieldCheck() {
if (this.value == "") {
this.style.backgroundColor = "#FFFF99";
this.focus();
}
else {
this.style.backgroundColor = "#FFFFFF";
}
}
|
1. |
if (allTags[i].className. indexOf("reqd") > -1) {
We're using a class attribute (of reqd) to decide on the fly when the onblur event handler should be used. Simply adding class="reqd" to an input tag triggers the event, instead of having to put the onblur handler on fields individually.
| 2. |
allTags[i].onblur = fieldCheck;
This event handler on the field causes the fieldCheck() function to be called whenever the user leaves a required field.
| | | 3. |
function fieldCheck() {
if (this.value == "") {
this.style.backgroundColor = "#FFFF99";
this.focus();
}
else {
this.style.backgroundColor = "#FFFFFF";
}
}
The fieldCheck() function checks to make sure that something (anything) was entered in the current field. If the field has no value, the field's background is colored pale yellow ( Figure 9.7), and the cursor gets put back into the form field with focus(). When the error is corrected, the background gets reset to white.
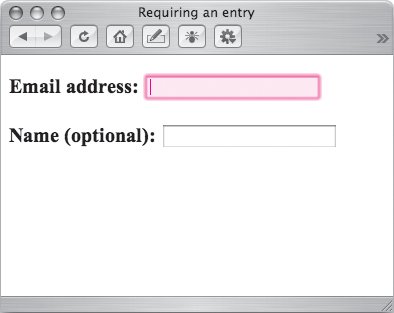
|
Tips
Both the onblur and onchange events are triggered when the user leaves a field after changing it. If the user leaves a field without changing it, just the onblur handler is triggered. Currently shipping versions of Firefox have a problem with focus(): even though you tell the browser to stay in a field, it doesn't. Changing the background color gives the user a visual cue that something's wrong, though, so they'll still know that there was a problem.
The onfocus event
Sometimes you'll have a form field on a page with data that you want to display as part of the form, without the user being able to modify that field. You can use the readonly HTML attribute to try to keep users out, but not all browsers support it. Scripts 9.17 and 9.18 show how to use the onfocus event to bump users right back out of this field, on the off chance they made it to where they shouldn't be.
Script 9.17. The HTML creates the form, which won't allow entries in the email field.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Forbidding an entry</title>
<script src="script09.js" type="text/javascript" language="javascript">
</script>
</head>
<body bgcolor="#FFFFFF">
<form action="#">
<h3>
Your message: <textarea rows="5" cols="30">Enter your message here</textarea><br
/><br />
Will be sent to: <input type="text" value="js6@javascriptworld.com"
readonly="readonly" />
</h3>
</form>
</body>
</html>
|
Script 9.18. Prevent wayward field entries with the onfocus handler in a form.
window.onload = initForm;
function initForm() {
var allTags = document.getElementsByTagName ("*");
for (var i=0; i<allTags.length; i++) {
if (allTags[i].readOnly) {
allTags[i].onfocus = function() {this.blur()};
}
}
}
|
allTags[i].onfocus = function() {this.blur()}; When the user attempts to enter this field, the focus (in this case the active field) will automatically be kicked right back out again (Figure 9.8). This happens because the onfocus event handler is set to call an anonymous function (one without a name) that does just one thing: call blur() on the current field, bouncing the user out.
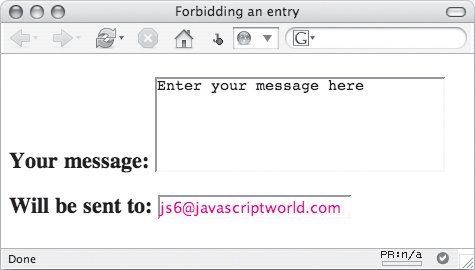
 |