Dragging and Dropping Page Elements
One of the nicest Ajax effects is the ability to drag-and-drop page elements to suit your preferences. You see this implemented on the personalized My Yahoo! and Google pages, which allow you to move around customizable modules (Figure 16.1).
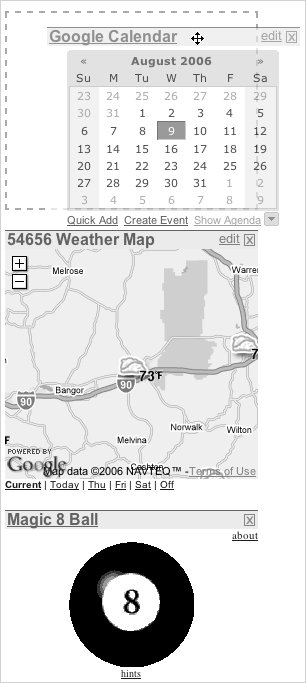
In this example, we've created a virtual light-table page for a Web-based slideshow (Figure 16.2). You can drag and drop the images into a particular order on the page. If this was a complete Web application, you could then click the Build it! button to create and play the slideshow in the order you chose. The HTML for the page is in Script 16.1, the CSS in Script 16.2, and the JavaScript in Script 16.3.
Script 16.1. This HTML creates the Slideshow Builder page and calls six YUI files and two of our own.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN">
<html>
<head>
<title>Drag and Drop</title>
<link type="text/css" rel="stylesheet" href="yui/screen.css" />
<link type="text/css" rel="stylesheet" href="script01.css" />
<script type="text/javascript" src="yui/yahoo.js"></script>
<script type="text/javascript" src="yui/event.js"></script>
<script type="text/javascript" src="yui/dom.js"></script>
<script type="text/javascript" src="yui/dragdrop.js"></script>
<script type="text/javascript" src="yui/DDList.js"></script>
<script type="text/javascript" src="script01.js"></script>
</head>
<body>
<h1>Slideshow Builder</h1>
<ul>
<li id="li0" class="sortList"><img src="images/IMG_1225.jpg" alt="IMG_1225.jpg"
width="128" height="96"/></li>
<li id="li1" class="sortList"><img src="images/IMG_1213.jpg" alt="IMG_1213.jpg"
width="128" height="96"/></li>
<li id="li2" class="sortList"><img src="images/IMG_1368.jpg" alt="IMG_1368.jpg"
width="128" height="96"/></li>
<li id="li3" class="sortList"><img src="images/IMG_1251.jpg" alt="IMG_1251.jpg"
width="128" height="96"/></li>
<li id="li4" class="sortList"><img src="images/IMG_1260.jpg" alt="IMG_1260.jpg"
width="128" height="96"/></li>
<li id="li5" class="sortList"><img src="images/IMG_1234.jpg" alt="IMG_1234.jpg"
width="128" height="96"/></li>
<li id="li6" class="sortList"><img src="images/IMG_1267.jpg" alt="IMG_1267.jpg"
width="128" height="96"/></li>
</ul>
<br clear="all" />
<div>
<form action="#">
<input id="build" type="submit" value="Build it!" />
<input id="revert" type="reset" value="Revert"/>
</form>
</div>
</body>
</html>
|
Script 16.2. Styling for Slideshow Builder is courtesy of this CSS.
h1, form {
margin-left: 40px;
}
li {
list-style-type: none;
float: left;
margin: 0 16px 16px 0;
}
.sortList {
width: 130px;
}
ul {
width: 600px;
}
|
Script 16.3. This JavaScript calls the YUI libraries and makes the drag and drop work.
YAHOO.DDApp = function() {
return {
init: function() {
for (j=0; j<7; j++) {
new YAHOO.example.DDList("li" + j);
}
YAHOO.util.DDM.mode = 1;
}
}
} ();
YAHOO.util.Event.addListener(window, "load", YAHOO.DDApp.init);
YAHOO.example.DDList.prototype.onDragOver = function(e, id) {
var el;
el = ("string" == typeof id) ? YAHOO.util.DDM.getElement(id): YAHOO.util.DDM
.getBestMatch(id).getEl();
var midY = YAHOO.util.DDM.getPosY(el) + (Math.floor(el.offsetTop / 2));
var midX = YAHOO.util.DDM.getPosX(el) + (Math.floor(el.offsetLeft / 2));
if (YAHOO.util.Event.getPageY(e) < midY) {
var el2 = this.getEl();
var p = el.parentNode;
p.insertBefore(el2, el);
}
if (YAHOO.util.Event.getPageX(e) < midX) {
var el2 = this.getEl();
var p = el.parentNode;
p.insertBefore(el2, el);
}
};
|
To enable drag and drop for page elements:
1. |
<link type="text/css" rel="stylesheet" href="yui/screen.css"/>
<link type="text/css" rel="stylesheet" href="script01.css" />
Most of the work done by YUI is handled almost entirely just by including links to its files in our HTML page. Here in Script 16.1, we've added a link to the YUI screen.css file, which is then followed by a link to our script01.css file. One of the handiest parts of the CSS cascade is that if there's something we don't like about Yahoo!'s styles, all we have to do is override a style in our own style sheet, and then link to it after theirs.
| | | 2. |
<script type="text/javascript" src="yui/yahoo.js"></script>
<script type="text/javascript" src="yui/event.js"></script>
<script type="text/javascript" src="yui/dom.js"></script>
<script type="text/javascript" src="yui/dragdrop.js"></script>
<script type="text/javascript" src="yui/DDList.js"></script>
<script type="text/javascript" src="script01.js"></script>
What went for CSS goes for JavaScript as well. However, there's a lot more JavaScript work going on behind the scenes, so we have to bring in several YUI JavaScript libraries. Here, we're bringing in yahoo.js, event.js, dom.js, dragdrop.js, and DDList.js before ending with our own script01.js.
What's in each of those files? One of the best parts of using a toolkit is that you don't have to learn all the detailsfollow Yahoo!'s examples and directions, and your code should work. One thing to always remember, though: you need to start off with Yahoo!'s yahoo.js file, as it sets up some global variables used by all of Yahoo!'s components.
| 3. |
<li id="li0" class="sortList"> <img src="images/IMG_1225.jpg" alt="IMG_1225.jpg"
width="128" height="96"/></li>
Here's one of the images on our page. It's inside a list tag, because we're using YUI's drag-and-drop list handler (see DDList.js, above) to handle our dragging and dropping needs.
| | | 4. |
li {
list-style-type: none;
float: left;
margin: 0 16px 16px 0;
}
Inside Script 16.2, we set the list style to not display as a list. While it needs to be one internally, there's no reason for it to look like one on the screen.
| 5. |
YAHOO.DDApp = function() {
return {
init: function() {
for (j=0; j<7; j++) {
new YAHOO.example.DDList ("li" + j);
}
YAHOO.util.DDM.mode = 1;
}
}
} ();
The code in Script 16.3 may look a little different than what you've seen previously in this book, but it's all a matter of coding style. Here, we're setting up some things to happen when the page first loads: calling YAHOO.example.DDList() to set up our list items, and setting YAHOO.util.DDM.mode to pick one of Yahoo!'s drag-and-drop styles. You'll notice that all of the functions and variables start with YAHOO; that's to allow them to coexist with other code and be sure that neither steps on or overwrites the other.
| | | 6. |
YAHOO.example.DDList.prototype. onDragOver = function(e, id) {
Once you learn how the Yahoo! naming style works, though, you can use that to your advantage. The method onDragOver is normally set deep within the DDList.js routinesbut we want to change how it works slightly. To do that, we don't need to step on Yahoo!'s code at all; instead, we just create our own version and put it into our own external .js file. Because ours is brought in last, we know that when it comes time to run this function, our version is the one that will be called.
|
Tips
In this example, the Build it! button is non-functional; all that works is the drag-and-drop capability. For examples of building slideshows, see Chapters 4 and 14. You should also take the time to check out the YUI Library CSS Tools, which are on the same page as the rest of the YUI Library. The CSS Tools include the Grids CSS component, which is a suite of Web page templates that allow you to create grids of one to four columns in each template. This lets you to create more than 100 fully standards-compliant page layouts, based on CSS, with a CSS library that is less than 2K in size. There are also the Fonts CSS and CSS Reset components, which give you greater control over font display and HTML rendering, respectively. When you download the YUI files, you'll find that they're grouped into various directories depending on what task they're associated with. You don't have to keep them that way, thoughyou can move them around in whatever way works best for your own style. In this chapter, we've moved them all into a yui directory of their own, one level below our HTML, CSS, and script pages.
The Yahoo! folks want you to use their toolkit, and they've created a few different places where you can learn about the package and interact with the growing community of users and developers that Yahoo! is building. Check out these resources:
The main Yahoo! User Interface Library page (developer.yahoo.com/yui/) has links to all of the components in the library, with extensive documentation, sample code, and running examples. This should be your first stop. The YUI developers have a weblog where they explain how to use the components and highlight innovative uses they've run across. You'll find the blog at yuiblog.com. If you need a bit more interactivity, there's a Yahoo! Group that allows you to ask and answer questions about YUI. Like all Yahoo! Groups, you can read the discussions on the Web or receive them in your email program as a mailing list. The address for the group is groups.yahoo.com/group/ydn-javascript/.
|
Back in the late 1990s, when Dynamic HTML was the latest rage, there were a variety of DHTML toolkits people wrote and made available. Some of the best were (in effect) written by a couple of guys in a garage somewhere. When the dot-com boom dot-bombed, the toolkits' authors had to get day jobs, and the packages were abandoned and not maintained. So when we looked for Ajax toolkits for this book, we were a bit wary.
We've chosen to discuss the Yahoo! User Interface Library in this chapter, because it is documented, high quality, open-source, and actively supported by a large company, which makes it more likely to stay available for the lifetime of this book. But there are many other good Ajax toolkits available. There are even sites that rate the different toolkits; at press time, our favorite was on the Musings from Mars weblog, at www.musingsfrommars.org/2006/03/ajax-dhtml-library-scorecard.html.
We think that the most important thing you should look for in a toolkit is that it does a great job of supporting Web standards. That means that it should fully support the most popular browsers, cross-platform. To us, that list includes Internet Explorer 6 and 7, Firefox for Windows and Mac, Safari for Mac, and Opera for Windows and Mac. It's also important that the toolkit be thoroughly debugged and that it have good documentation.
We suggest that you also take a look at these other toolkits, all of which are popular, are well-supported, have great demos on their sites so you can see if they meet your needs, and are well-documented:
If you are working with Adobe Flex and Flash and need an interface between JavaScript and ActionScript, take a look at the Flex-Ajax Bridge (FABridge), an open-source library from Adobe. To find it, go to the Adobe Web site and do a search, or look for the link on the Resources page at www.javascriptworld.com.
Finally, we should mention the Atlas framework from Microsoft (atlas.asp.net). It is heavily tied to ASP.NET server-side development. We can only recommend it if you plan to deploy your Web applications only to Microsoft browsers running on Windows, as its cross-platform capabilities, at press time at least, were poor.
|
|